记录一道计算几何题。
接触计算几何题不多,这个题挺典型也挺有意思的,记录一下。
题意
求一个椭圆与一个三角形相交部分的面积。
整体思路
几何变换,简化椭圆方程。
数值积分,将交集区域的面积近似为多个小矩形的面积之和。
几何变换
考虑将椭圆标准化为 $\dfrac{x^2}{a^2}+\dfrac{y^2}{b^2}=1$ 的形式,分为两步:平移、旋转。
平移:很简单,先求出新的原点坐标,即 A、B 中点坐标,将所有点减去该点坐标即可。
旋转:希望将 A 点旋转到 $x$ 轴负半轴作为左焦点,B 点旋转到 $x$ 轴正半轴作为右焦点。
计算逆时针旋转的角度 $\theta$,用 $2\pi$ 减去 OB 所在角度即可。
接着,利用旋转矩阵
$$
R(\theta)=\begin{bmatrix}
\cos\theta & -\sin\theta\\
\sin\theta & \cos\theta
\end{bmatrix}
$$
对每个点作旋转变换即可。
$$
\begin{bmatrix}
x’\\
y’
\end{bmatrix}
=\begin{bmatrix}
\cos\theta & -\sin\theta\\
\sin\theta & \cos\theta
\end{bmatrix}
\begin{bmatrix}
x\\
y
\end{bmatrix}=
\begin{bmatrix}
x\cos\theta - y\sin\theta\\
x\sin\theta+y\cos\theta
\end{bmatrix}
$$
数值积分
将交集区域的面积近似为多个小矩形的面积之和,对于每个 $x$ 值 $(-a\le x\le a)$,需要求出竖直线与椭圆相交的区间和与三角形相交的区间,取两个区间的交,作为小矩形的高。
- 与椭圆相交的区间为 $\left[-b\sqrt{1-\dfrac{x^2}{b^2}}, b\sqrt{1-\dfrac{x^2}{b^2}}\right]$。
- 与三角形相交的区间,可以用如下方式求得:
- 若三个顶点分布在竖直线左右两侧,那么有相交,否则无相交。
- 有相交时,不妨假设左侧有一点,右侧有两点,那么竖直线与三角形交于左侧一点与右侧两点的两条连线上,分别计算交点即可。
精度问题
$dx$ 的取值,过小容易 TLE,过大容易导致精度问题,估计一下时间复杂度,每个小矩形 $O(1)$,一共计算 $\dfrac{L}{dx}$ 次,$L=1e3$,取 $dx=1e-4$,整体约 $1e7$ ,在时限内且保证精度。
代码实现
1 |
|
有趣的是
你想去洛谷找题解的话,看到的还是这篇)
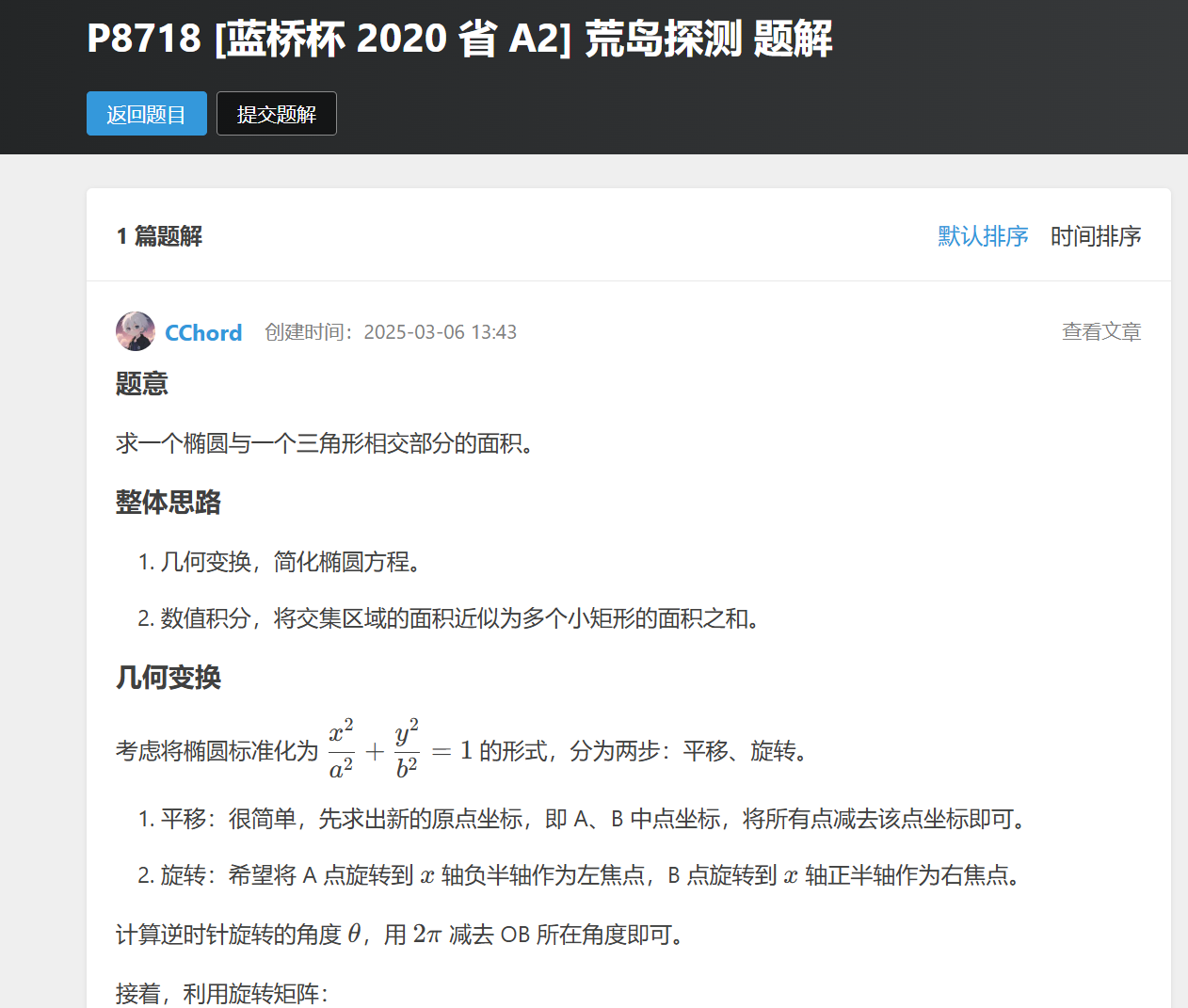